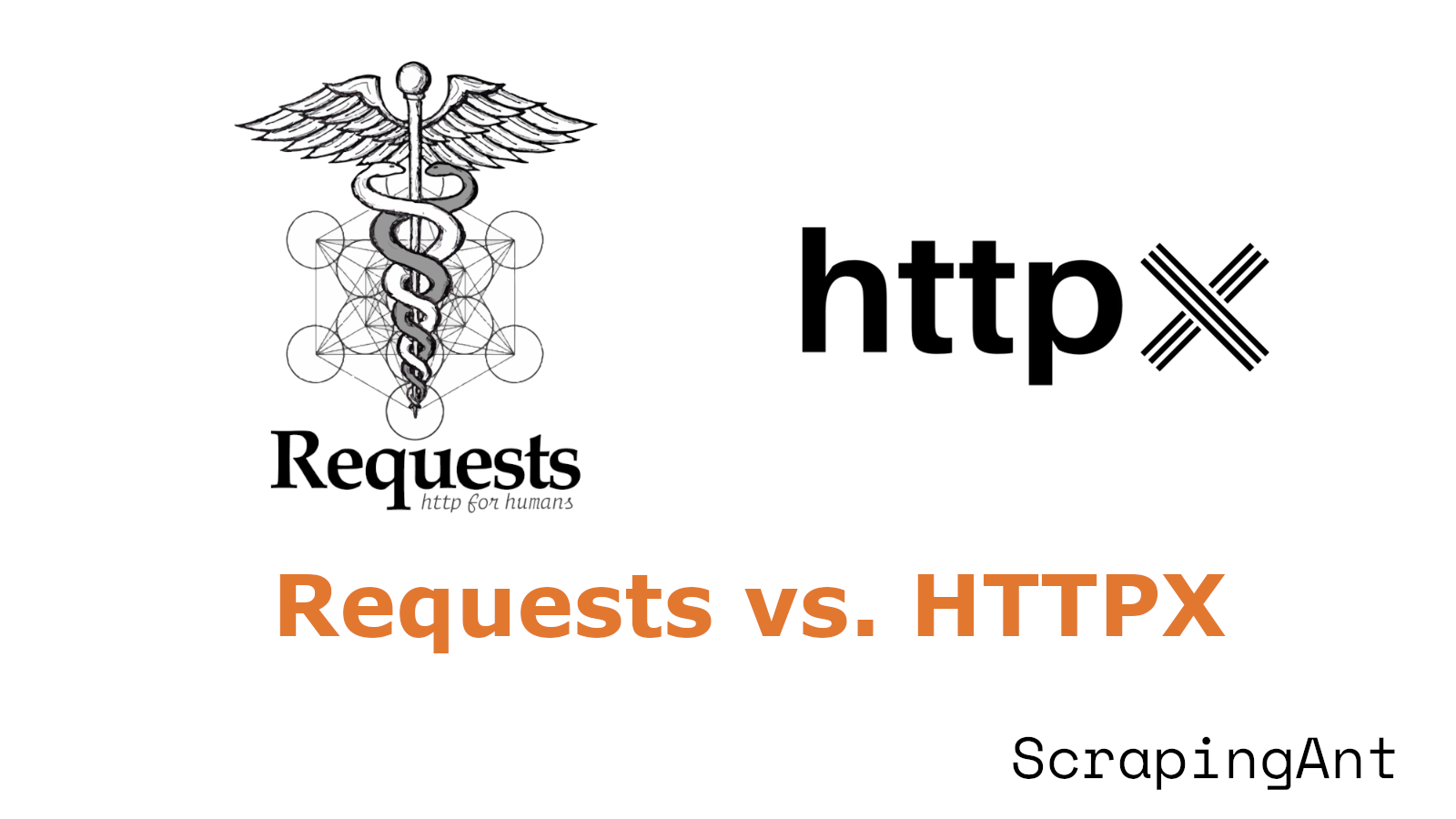
In the realm of Python development, making HTTP requests is a frequent task that requires efficient and reliable libraries. Two prominent libraries, Requests and HTTPX, have been widely adopted by developers for this purpose. Each library has its strengths and weaknesses, making the choice between them dependent on the specific requirements of the project. This research aims to provide a comprehensive comparison between Requests and HTTPX, considering various aspects such as asynchronous support, HTTP/2 compatibility, connection management, error handling, and performance metrics.
Requests, a well-established library, is celebrated for its simplicity and ease of use. It is often the go-to choice for developers who need to make straightforward, synchronous HTTP requests. However, its lack of native support for asynchronous operations and HTTP/2 can be a limitation for high-concurrency applications. On the other hand, HTTPX, a newer library, offers advanced features such as asynchronous support and HTTP/2, making it a more powerful tool for performance-critical applications.
This research will delve into the key feature comparisons and performance metrics of both libraries, providing detailed code examples and explanations. By examining these factors, developers can make an informed decision on which library best suits their needs. This comparison is supported by various benchmarks and source.