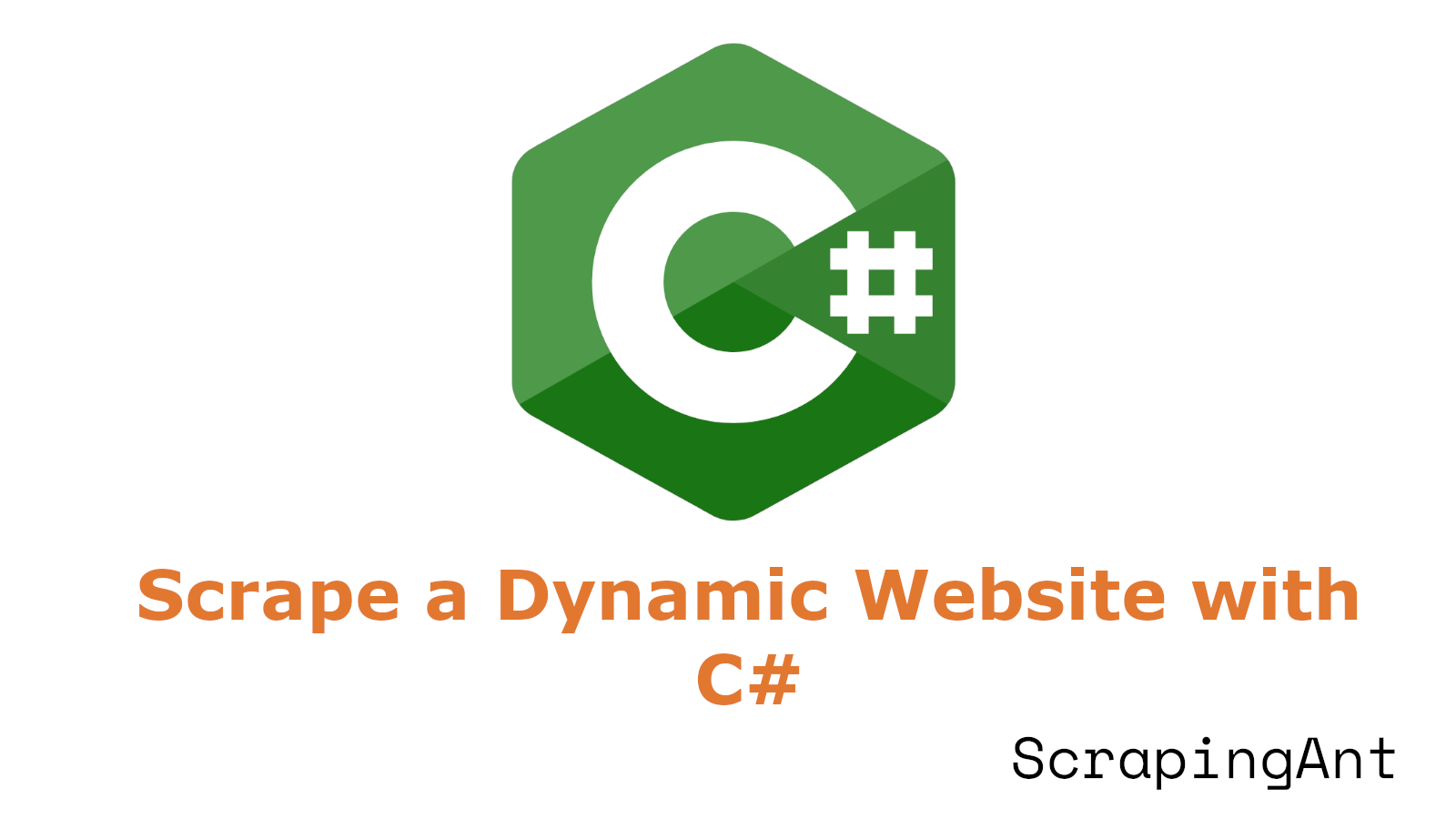
Dynamic websites have become increasingly prevalent due to their ability to deliver personalized and interactive content to users. Unlike static websites, which serve pre-built HTML pages, dynamic websites generate content on-the-fly based on user interactions, database queries, or real-time data. This dynamic nature is achieved through the use of server-side programming languages such as PHP, Ruby, and Python, as well as client-side JavaScript frameworks like React, Angular, and Vue.js.
Dynamic websites are characterized by asynchronous content loading, client-side rendering, real-time updates, personalized content, and complex DOM structures. These features enhance user experience but also introduce significant challenges for web scraping. Traditional scraping tools that rely on static HTML parsing often fall short when dealing with dynamic websites, necessitating the use of more sophisticated methods and tools.
To effectively scrape dynamic websites using C#, developers must employ specialized tools such as Selenium WebDriver and PuppeteerSharp, which can interact with web pages as if they were real users, executing JavaScript and waiting for content to load. These tools, along with proper wait mechanisms and dynamic element location strategies, enable the extraction of data from even the most complex and interactive web applications.